Global Mapper Python reference¶
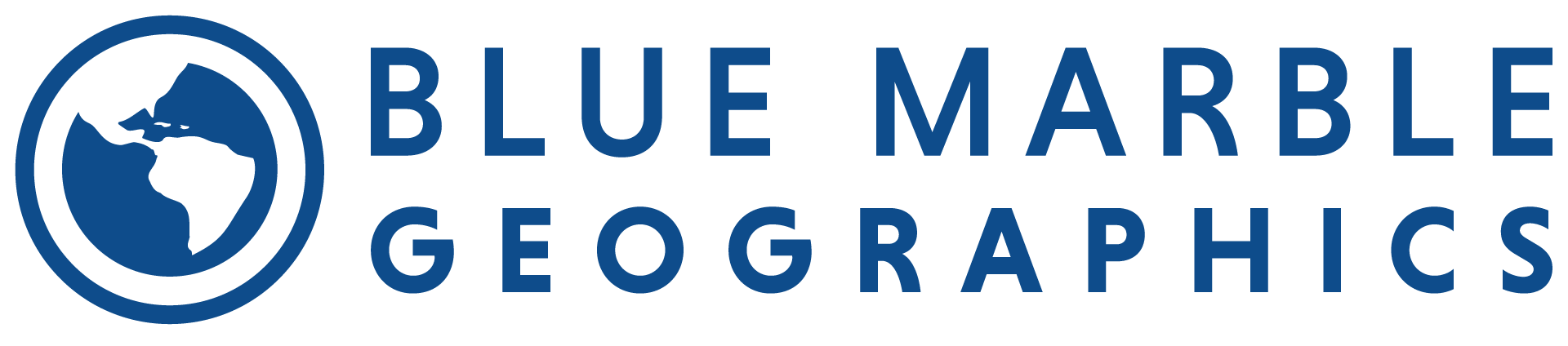
Python integration in Global Mapper enables users of the program to write scripts to automate Global Mapper workflows using the Python programming language. Many functions available in the user interface of Global Mapper are able to be accessed and run through a Python script. Available operations range from changing program settings, importing and exporting data, vector/terrain/lidar/image analysis, and many others.
Python script files (*.py) can be run in Global Mapper through the Script Editor tool or executed via the command line or in a Python environment.
Contents:
- Installation and Setup Guides
- Functions
- Layer Load Functions
- Display Options/Drawing Functions
- Coordinate Conversion/Measurement Functions
- Export Functions
- Raster/Elevation Layer Query Functions
- Vector Layer Query Functions
- Lidar Functions
- Vector Layer Edit Functions
- Vector Drawing Style Functions
- Vector Feature Filtering Functions
- 3D View Functions
- Surface Analysis Functions
- Map Catalog Functions
- Custom Layer and Feature Creation Functions
- Spatial Operations
- Utility Functions
- globalmapper Base Classes
- Constants
- Types for Surface Analysis
- Types for Display and Drawing
- Types for Exporting
- Types for Lidar Functions
- Types for Layer Loading Functions
- Types for Measurement Functions and Units
- Types for Raster Data and Operations
- Types for Spatial Operations
- Types for Utility Purposes
- Types for Vector Data and Operations
- Code Examples and Explanations
- Opening and Closing a Layer
- C++-Style Arrays for GM Types in Python
- Converting a Pointer to an Array or List
- Global Mapper Ints: *_t32, uint32, and others
- Creating and Setting Projections
- Selecting a File to Open in GlobalMapper
- Scripting Example Using RunScript
- Handling Text Encodings
- Full code examples